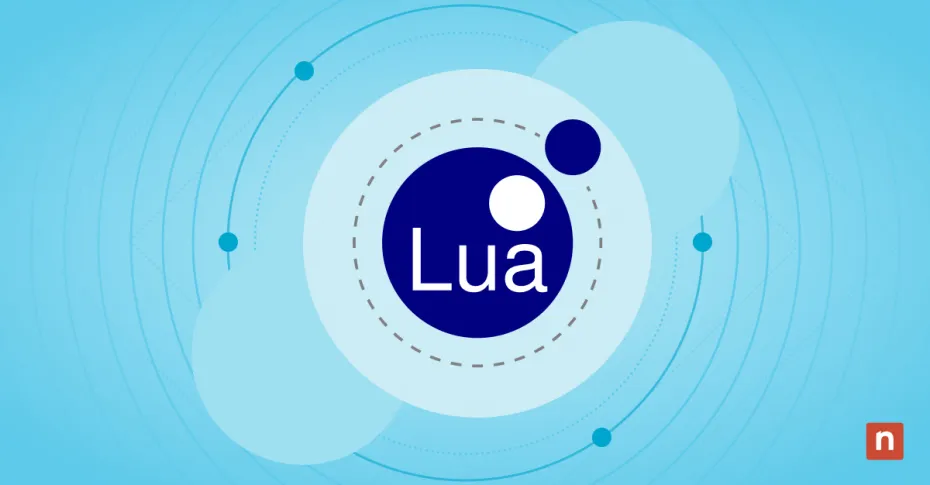
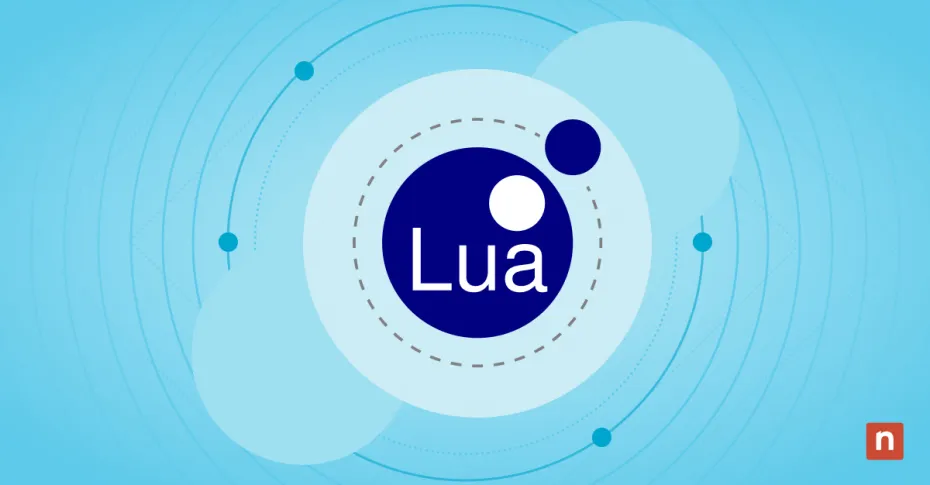
Hello World#
foo.lua
print('Hello World!')
luashell
lua foo.lua
shellComment#
-- comment
--[[
multi-line
comment
]]
luaVariables#
num = 42
fnum = 42.2
b = true
s = 'string'
t = "This is a immutable string."
u = [[ This is a multi-line
string. ]]
t = nil -- undefines t
luanil
表示 “无” 或 “没有值”, Lua 有内存回收机制
thisIsGlobal = 5 -- global by default
local localVal = 6
luafoo = undefinedVal -- foo -> nil
space = ' '
io.write('Hello' .. space .. 'World!')
lua- 变量默认是全局的
- 未定义变量的值是
nil
, 并不会产生 Error ..
连接字符串
Flow control#
while num < 50 do
num = num + 1 -- no += or ++
end
if num > 40 then
print('over 40')
elseif s ~= 'string' then
io.write('less than 40')
else
local line = io.read()
print('You typed: ' .. line)
end
luaaBoolValue = false
-- This is similar to the a?b:c operator in C/js:
aBoolValue = aBoolValue and 'yes' or 'no' --> 'no'
if not aBoolValue then print('it was false') end
lua- 只有
nil
和false
是假值, 其他如0
或''
都是真值
karlSum = 0
for i = 1, 100 do -- includes 1 and 100
karlSum = karlSum + i
end
-- begin, end[, step]
fredSum = 0
for j = 100, 1, -1 do fredSum = fredSum + j end
lua- for 循环包括两端
repeat
print('the way of the future')
num = num - 1
until num == 0
luaFunctions#
function fib(n)
if n < 2 then return 1 end
return fib(n - 2) + fib(n - 1)
end
luafunction makeCounter()
local count = 0 -- 局部变量,闭包捕获
return function()
count = count + 1
return count
end
end
local counter = makeCounter() -- 返回一个闭包
print(counter()) -- 输出 1
print(counter()) -- 输出 2
print(counter()) -- 输出 3
function adder(x)
return function (y) return x + y end
end
a1 = adder(9)
a2 = adder(36)
print(a1(16)) --> 25
print(a2(64)) --> 100
lua- 这里涉及到了闭包 (closure) 和匿名函数 (anonymous function)
- 闭包延长变量生命周期, 例如
makeCounter
函数执行完毕,count
变量仍然能被闭包引用和修改
m, n, j, k = 1, 2, 3 -- k = nil
x, y, z = 1, 2, 3, 4 -- 4 is thrown away.
function bar(a, b, c)
print(a, b, c)
return 4, 8, 15, 16, 23, 42
end
lua- Returns, func calls, and assignments all work with lists that may be mismatched in length
- Unmatched receivers are nil
- Unmatched senders are discarded
print("Hello")
print "Hello" -- works fine
lua- Calls with one string param don’t need parens
local function g(x) return math.sin(x) end
local g; g = function (x) return math.sin(x) end
lua- Functions are first-class, may be local/global
Tables#
t = {key1 = 'value1', key2 = false}
print(t.key1)
t.newKey = {}
t.key2 = nil
luau = {['@!#'] = 'Hello', [{}] = 1729, [6.28] = 'World'}
print(u['@!#'] .. ' ' .. u[6.28])
x = u[{}] -- x = nil
lua-- A one-table-param function call needs no parens:
function h(x) print(x.key1) end
h{key1 = 'Sonmi~451'} -- Prints 'Sonmi~451'.
for key, val in pairs(u) do -- Table iteration.
print(key, val)
end
luax = 42 -- 创建一个全局变量 x
print(_G.x) -- 输出 42
_G.y = "Hello" -- 创建一个全局变量 y
print(y) -- 输出 "Hello"
local varName = "x"
print(_G[varName]) -- 输出 42
print(_G.varName) -- 输出 nil
print(_G['_G'] == _G) -- true
luav = {'value1', 'value2', 1.21, 'gigawatts'}
for i = 1, #v do -- #v -> size of v
print(v[i]) -- Indices start at 1 !! SO CRAZY!
end
lua- 作为 list 使用时, 是从 1 开始计数的
t = {}
function t:func(x, y)
self.x = x
self.y = y
end
t:func(1, 1)
print(t.x) -- 1
-- same as
function t.func(self, x, y)
self.x = x
self.y = y
end
t.func(t, 1, 1)
lua关于 Class-like tables 的内容此处省略.
Moudles#
-- mod.lua
local M = {}
local function sayMyName()
print('Hrunkner')
end
function M.sayHello()
print('Why hello there')
sayMyName()
end
return M
lua-- Another file can use mod.lua's functionality:
local mod = require('mod') -- Run the file mod.lua. (run once)
local mod2 = require('mod') -- mod2 = mod, but not run mod.lua (cached)
--[[ require acts like:
local mod = (function ()
<contents of mod.lua>
end)()
--]]
mod.sayHello() -- Prints: Why hello there Hrunkner
-- mod.sayMyName() -- error
lua-- dofile is like require without caching:
dofile('mod2.lua') --> Hi!
dofile('mod2.lua') --> Hi! (runs it again)
-- loadfile loads a lua file but doesn't run it yet.
f = loadfile('mod2.lua') -- Call f() to run it.
-- load is loadfile for strings.
-- (loadstring is deprecated, use load instead)
g = load('print(343)') -- Returns a function.
g() -- Prints out 343; nothing printed before now.
luapackage.path = package.path .. ';../?.lua'
require("folder.subfolder.file")
luarequire
将.
替换成 directory separator (/
on Unix systems)- lua 在
package.path
中搜索文件, 其中包含了./?.lua
, 加载过的文件将被加入到package.loaded